How to use Dockerfile to create Docker Image
Dockerfiles are text files that contain instructions for building Docker images. They define the environment inside a container and specify what needs to be installed, configured, and executed. Here’s a breakdown of the components typically found in a Dockerfile:
- Base Image: The
FROM
instruction specifies the base image upon which the new image will be built. It’s the starting point for your image.Example:
FROM ubuntu:latest
2. Environment Setup: You can use ENV
to set environment variables that will be available during the build process and in the container. Example:
ENV NODE_ENV=production
3. Working Directory: WORKDIR
sets the working directory inside the container where subsequent instructions will be executed.
WORKDIR /app
4. Copy Files: COPY
copies files or directories from the host machine into the image at a specified destination. Example:
COPY . /app
5. Install Dependencies: Use RUN
to execute commands within the image during the build process. This is commonly used to install dependencies.Example:
RUN apt-get update && apt-get install -y python3
6. Expose Ports: EXPOSE
documents which ports the container will listen on at runtime.Example:
EXPOSE 8080
So, Here we are writing a Dockerfile to run the nginx web server just to deploy a simple “Hello world” HTML page.
1. Write a Dockerfile
Create a file named Dockerfile
in your project directory.
# Use the official Nginx image based on Ubuntu
FROM nginx:latest
# Copy the index.html file into the default Nginx html directory
COPY index.html /usr/share/nginx/html
# Expose port 80 to allow incoming connections
EXPOSE 80
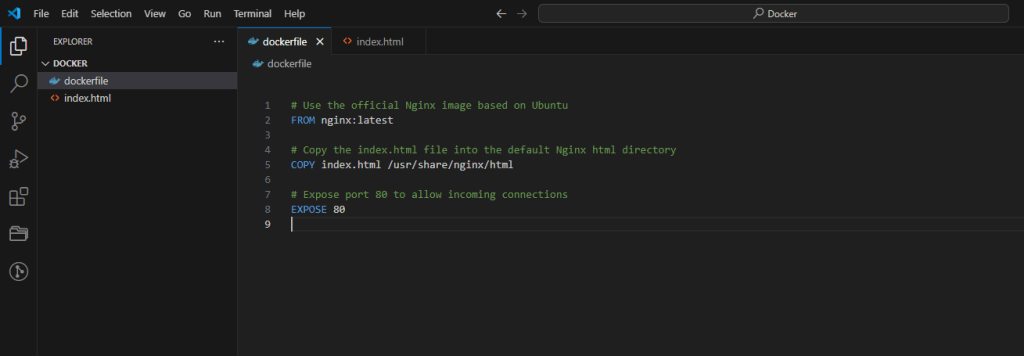
2. Build the Docker Image
Navigate to the directory containing your Dockerfile (cd path/to/your/project
) and run the following command to build the Docker image:
docker build -t nginx .
This command builds the Docker image with the tag ‘nginx’

3. Run a Container
After the image is built, you can run a container based on it using the following command:
docker run -d -p 8080:80 nginx

This command runs a container based on the nginx image, exposing port 80 of the container to port 8080 on your host machine. We can then access your blog by navigating to http://localhost:8080
in our web browser.
In summary, Dockerfiles are the cornerstone of Docker containerization, offering a structured approach to defining container environments. By encapsulating setup, dependencies, and execution instructions, Dockerfiles streamline the creation of lightweight and portable Docker images. Happy Coding.